本部分主要笔记Guava之Collections的用法
Immutable Collections
不可变的对象有很多好处:
- 不受信任的库可以安全使用。
- 线程安全:可以被许多线程使用,没有竞争条件的风险。
- 不需要支持变异,并且可以通过该假设节省时间和空间。所有不可变的集合实现都比它们可变的兄弟节点更具内存效率
- 可以用作常量,期望它将保持固定。
防御性编程(defensive programming technique)
Guava 为JDK里面的各个几何类都提供了一个不可变的版本
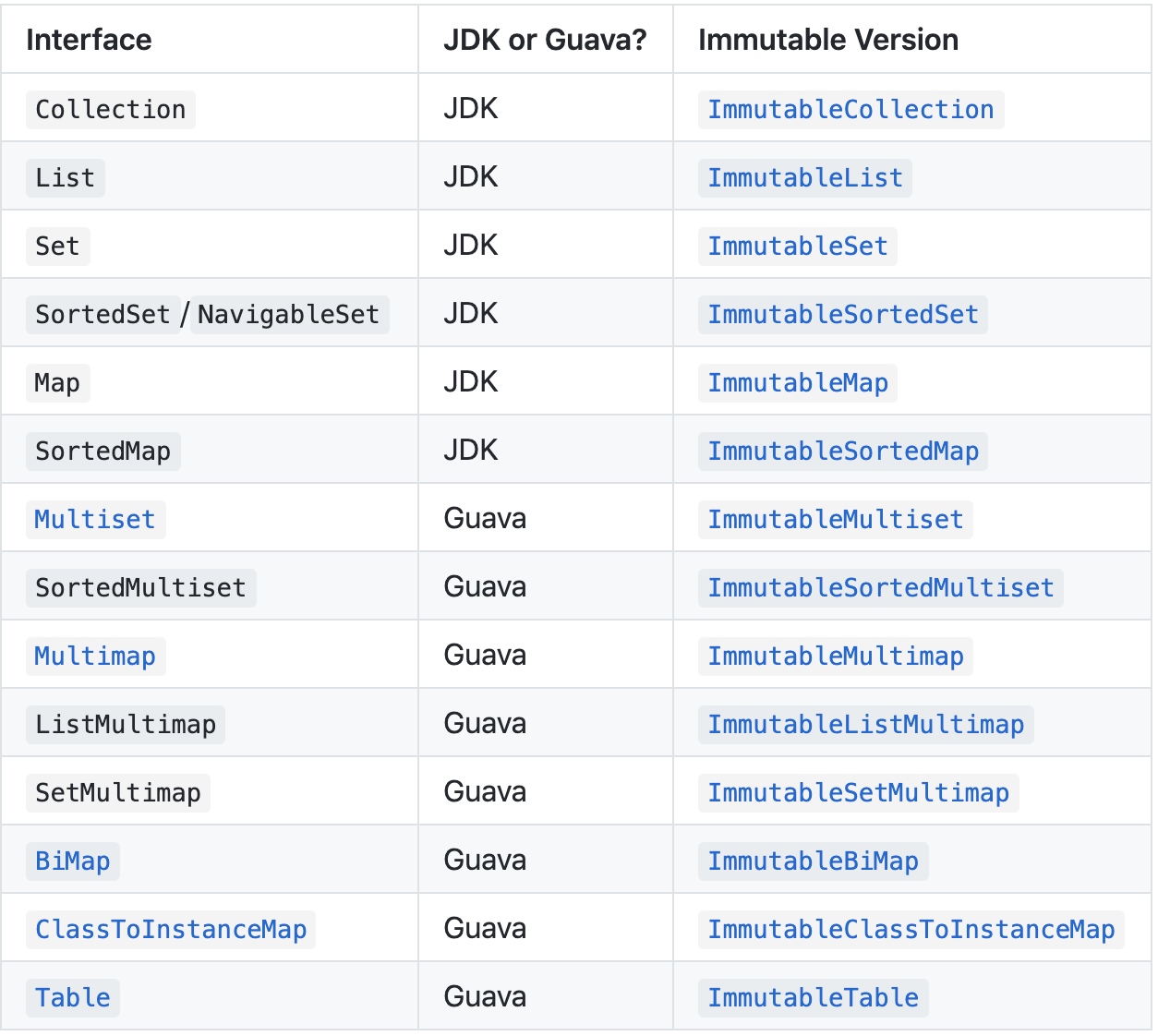
3种创建方式
- 使用copyOf()
使用of():
1
| ImmutableSet.of("a", "b", "c", "a", "d", "b")
|
Builder
1 2 3 4 5
| public static final ImmutableSet<Color> GOOGLE_COLORS = ImmutableSet.<Color>builder() .addAll(WEBSAFE_COLORS) .add(new Color(0, 191, 255)) .build();
|
New Collection Types
Guava在JDK的基础上,重新定义了几个集合类型
Multiset
我们知道Set集合取值是没有重复的,Multiset和Set的区别在于Multiset是可以存重复的值的
1 2 3 4 5 6 7 8 9 10 11 12 13
| public void test2() { Multiset<String> multiset = HashMultiset.create(); multiset.add("apple", 2); multiset.add("orange");
System.out.println(multiset.count("apple"));
System.out.println(multiset.setCount("apple", 2, 5)); System.out.println(multiset.count("apple")); System.out.println(multiset.setCount("apple", 2, 6)); System.out.println(multiset.count("apple")); }
|
从上面的示例可以看出Multiset是一个很好用的计数容器。
Multimap
这个集合对象也特别有意思,它和Map不同,Map结构是一个K对应一个V,而Mutilmap是一个K对应多个V
1 2 3 4 5 6 7 8 9
| public void test3() { Multimap<String, String> multimap = HashMultimap.create(); multimap.put("apple", "red"); multimap.put("apple", "green"); multimap.put("apple", "yellow"); multimap.put("orange", "orange"); Collection<String> attrs = multimap.get("apple"); attrs.forEach(System.out::println); }
|
怎么样,挺有意思吧?同时Multimap还提供了一组视图方法,用来转换multimap对象的数据到其它的数据结构视图,这个就不穷举说明了,看文档
Muiltumap提供了多种实现
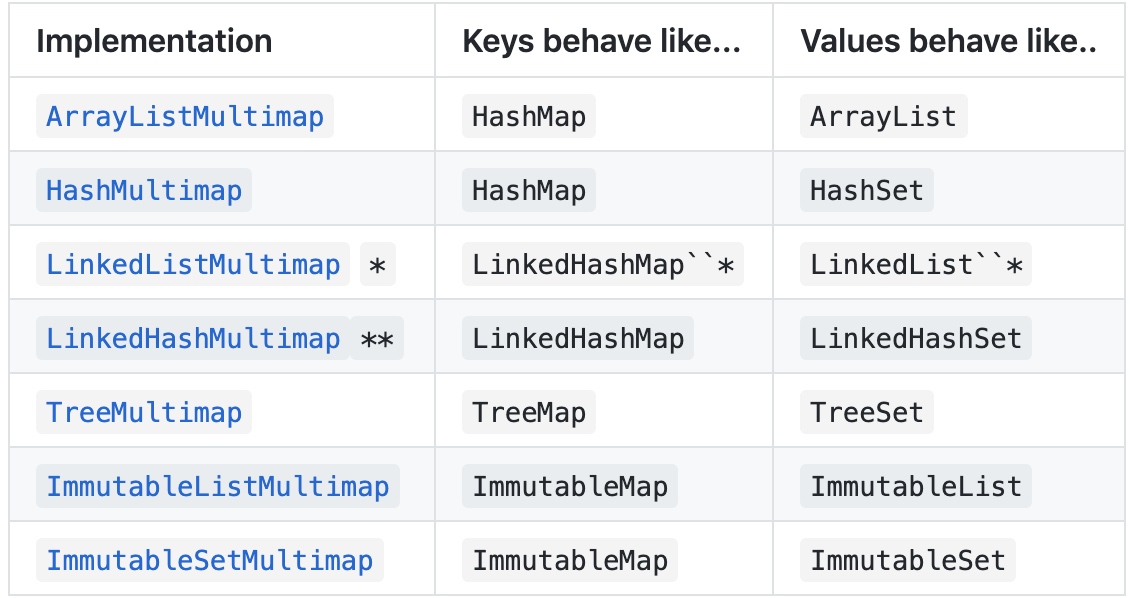
BiMap
BiMap在Map的基础上,提供了一个reverse()方法,可以将Map的key和value值进行对掉。
1 2 3 4 5 6 7 8
| public void test4() { BiMap<Integer, String> biMap = HashBiMap.create(); biMap.put(1, "apple"); biMap.put(2, "guava"); biMap.put(3, "kiwi"); BiMap<String, Integer> biMapReverse = biMap.inverse(); assertEquals(1, biMapReverse.get("apple")); }
|
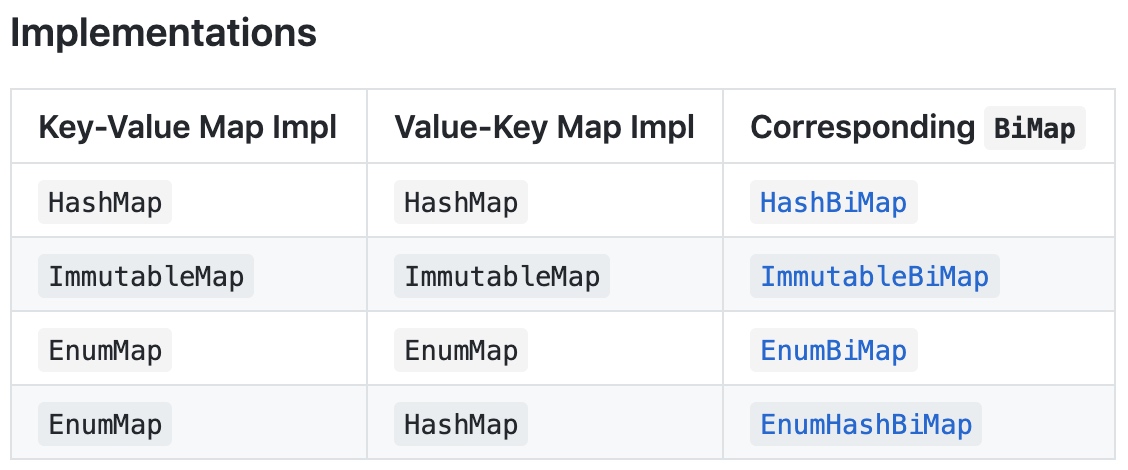
Table
Table用来记录一个坐标的值
1 2 3 4 5 6 7 8 9
| public void test5() { Table<String, String, Integer> table = HashBasedTable.create(); table.put("1", "1", 11); table.put("1", "2", 12); table.put("1", "3", 13); table.put("2", "1", 21); table.put("2", "2", 22); assertEquals(12, table.get("1", "2")); }
|
ClassToInstanceMap
ClassToInstanceMap提供了K为class,V为值的map类型,emmm,没想到应用场景
1 2 3 4 5
| public void test6() { ClassToInstanceMap<Number> numberDefaults = MutableClassToInstanceMap.create(); numberDefaults.putInstance(Integer.class, Integer.valueOf(1)); numberDefaults.putInstance(Double.class, Double.valueOf(1)); }
|
RangeSet
RangeSet用来处理一些连续的非空的range,当添加一个range到一个RangeSet之后,任何有连续的range将被自动合并,而空的range将被自动去除
1 2 3 4 5 6 7 8 9 10
| public void test7() { RangeSet rangeSet = TreeRangeSet.create(); rangeSet.add(Range.closed(0, 10));
rangeSet.add(Range.closedOpen(10, 15));
rangeSet.add(Range.closedOpen(16, 18));
System.out.println(rangeSet); }
|
RangeMap
和RangeSet相比,RangeMap将Range作为了一个key,每一个Range对应一个值。
1 2 3 4 5 6 7
| public void test8() { RangeMap rangeMap = TreeRangeMap.create(); rangeMap.put(Range.closed(5, 10), "apple"); rangeMap.put(Range.closed(8, 12), "banana"); System.out.println(rangeMap); }
|
Collection Utilities
Guava为一些集合接口开发了一组工具类
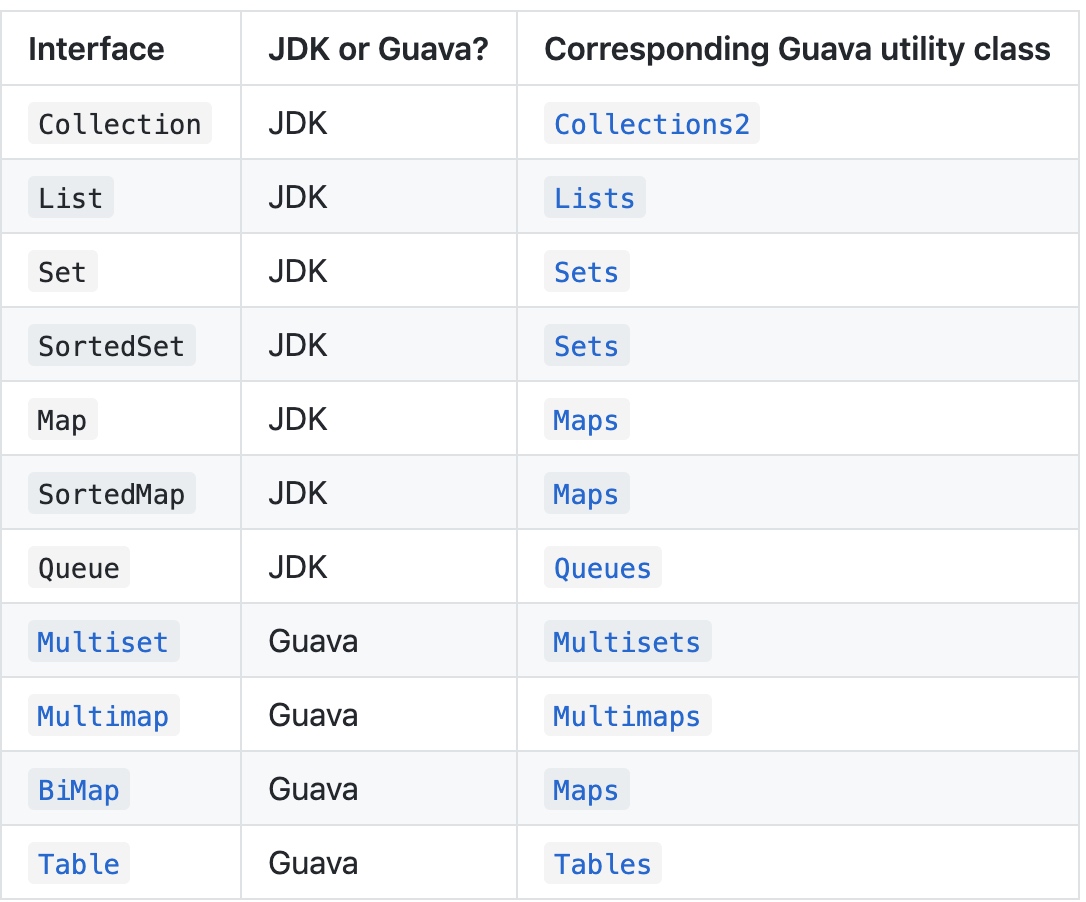
Extension Utilities
这个以后再看